I recently received a Feature Request to add functionality to a PowerShell script that would display the hardware and OS information of the machine the script was running on. Below you can find the PowerShell Function I created for this task, as well as some information on what each part of the function does.
function GetResources{
$computername = $env:COMPUTERNAME
$ProductName = (Get-ItemProperty 'HKLM:\SOFTWARE\Microsoft\Windows NT\CurrentVersion' -Name ProductName).ProductName
$cpu = Get-WmiObject -class Win32_Processor
$cpuCores = $cpu.numberofcores
$memory = Get-WmiObject -class "win32_physicalmemory" -namespace "root\CIMV2"
$disks = get-wmiobject -class "Win32_LogicalDisk" -Filter "DriveType = '3'" | Select-Object -Property DeviceID, @{L="Size (GB)";E={"{0:N2}" -f ($_.Size/1GB)}}
Write-Host -ForeGroundColor green -BackgroundColor black "Name:" "$computername"
Write-Host -ForeGroundColor green -BackgroundColor black "OS:" $ProductName
Write-Host -ForeGroundColor green -BackgroundColor black "RAM:"($memory.Capacity/1GB)"GB" -ErrorAction SilentlyContinue
Write-Host -ForeGroundColor green -BackgroundColor black "Cores:" $cpuCores
Write-Host -ForeGroundColor green -BackgroundColor black "Disks:"
$disks | Format-Table | Out-String | Write-Host -ForeGroundColor green -BackgroundColor black
}
GetResources
Most of this is pretty self explanatory, but it is worth mentioning the following:
$cpu = Get-WmiObject -class Win32_Processor
- This variable calls the WMI Object Class Win32_Processor. You’ll notice that we don’t actually output this query anywhere. That would be a mess! Even though we don’t output this raw data, querying this information is required to obtain the processor information and to pull out what we want later.
$cpuCores = $cpu.numberofcores
- This is where that previous query comes into play. Here we have created a variable called “$cpuCores”, which pulls out the specific number of cores from the Win32_Processor Object. It’s worth noting that this query will list the number of cores on each socket, so for machines with multiple CPUs, you’ll want to add the numbers together. I have not yet addressed this in my script as it is mostly an aesthetic thing.
$disks = get-wmiobject -class "Win32_LogicalDisk" -Filter "DriveType = '3'" | Select-Object -Property DeviceID, @{L="Size (GB)";E={"{0:N2}" -f
- This fun little command here gets the WMI Object Class Win32_LogicalDisk. This command has a few parts, so I’ll break this one down.
$disks = get-wmiobject -class "Win32_LogicalDisk"
- Much like the Win32_Processor query from earlier, we’re going to call this to have the information loaded that we’re going to want to query after the pipe in this line.
-Filter "DriveType = '3'"
- Here we’re applying a filter to the type of drive that we’re looking for. Different types of drives have different numbers. In this case, I’m only concerned about attached local disks. You can change this to whatever you want, depending on your unique use case. The different drive types can be found below:
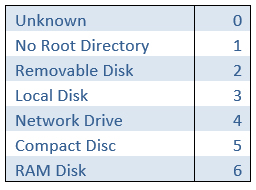
| Select-Object -Property DeviceID,
- Here we’ve piped out the query to “Select-Object” to pick out what we want for the table we’re going to create. Speaking of pipes, if you’re thinking to yourself, “couldn’t that have been two variables again? Why wasn’t it?” You’re thinking along the right lines. It could have been, but I just put it all on one line to save space. We could have split it into two variables and lines like earlier, but I didn’t feel like that was needed here.
@{L="Size (GB)";E={"{0:N2}" -f ($_.Size/1GB)}}
- Now we have this crazy looking thing. This is us creating labels for the table we’re going to create later and telling PowerShell to convert the disk size into a more readable GB format. The “@” symbol is a splat and is used for “Splatting”. Splatting is a method of passing a collection of parameter values to a command as a unit. To find out more about Splatting and how it works, read this Microsoft Article.
Write-Host -ForeGroundColor green -BackgroundColor black "RAM:"($memory.Capacity/1GB)"GB" -ErrorAction SilentlyContinue
- The next section of interest is here, where we write out the information we’ve collected and formatted to the console for viewing. You’ll see the variable “$memory.Capacity/1GB” this is telling PowerShell to take the Capacity out of the Memory Object Class that we queried in the script and display it in GBs. PowerShell accomplishes this by dividing the number it receives by 1 GB, which is a built in unit that does not need to be specified to be used. This gives us a more immediately human readable number that looks pretty. Also note the “-ErrorAction SilentlyContinue” tagged onto the end. This provides some error handling because I have found that some systems with huge amounts of memory do not display properly when dividing by 1 GB. This just ensures that the script continues on without injecting an obtrusive red error into the console.
$disks | Format-Table | Out-String | Write-Host -ForeGroundColor green -BackgroundColor black
- This is the last part of interest and the end of our function! Here we call the “$disks” variable and output it to “Format-Table”. From there, we pipe it into “Out-String”, which converts objects that Powershell manages into an array of strings. This is needed to pipe to “Write-Host”, which is what will output the nicely formatted table we created earlier. I’ve also tagged on some color formatting using “-ForeGroundColor green” and “-BackgroundColor black”. You can make these whatever colors you’d like. They are mostly there because I like the aesthetic and I believe they help pull attention to important parts of the script output.
Now that everything has been put together, we can run the function and we should recieve a nicely formatted output that looks like this:
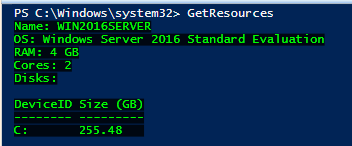
Using what you’ve learned above, you could add any number of things to the function to suit your individual use case. Items such as IP address, user name, groups, domain, etc, could be added easily.
I hope this helped out at least a couple of people! Happy scripting, and PowerShell ALL the things!
1 thought on “Powershell Function to Display Machine Hardware and Operating System Information”
Comments are closed.